mirror of
https://github.com/vale981/apollo-server
synced 2025-03-06 02:01:40 -05:00
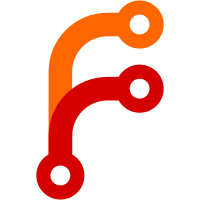
* Added fixes to apolloAzureFunctions.js and sample functions for the GraphQL API and GraphiQL * Added issue and pr details to changelog * Maintain use of `context.done()`, but use `isRaw` instead. Per the Azure documentation regarding the response object available at: https://docs.microsoft.com/en-us/azure/azure-functions/functions-reference-node#response-object * Switch Azure example to use CommonJS rather than native ESM exports.
70 lines
1.8 KiB
Markdown
Executable file
70 lines
1.8 KiB
Markdown
Executable file
---
|
|
title: Azure Functions
|
|
description: Setting up Apollo Server with Azure Functions
|
|
---
|
|
|
|
[](https://badge.fury.io/js/apollo-server-core) [](https://travis-ci.org/apollographql/apollo-server) [](https://coveralls.io/github/apollographql/apollo-server?branch=master) [](https://www.apollographql.com/#slack)
|
|
|
|
This is the Azure Functions integration for the Apollo community GraphQL Server. [Read the docs.](https://www.apollographql.com/docs/apollo-server/)
|
|
|
|
## Sample Code
|
|
|
|
### GraphQL:
|
|
|
|
```javascript
|
|
const { graphqlAzureFunctions } = require('apollo-server-azure-functions');
|
|
const { makeExecutableSchema } = require('graphql-tools');
|
|
|
|
const typeDefs = `
|
|
type Random {
|
|
id: Int!
|
|
rand: String
|
|
}
|
|
|
|
type Query {
|
|
rands: [Random]
|
|
rand(id: Int!): Random
|
|
}
|
|
`;
|
|
|
|
const rands = [{ id: 1, rand: 'random' }, { id: 2, rand: 'modnar' }];
|
|
|
|
const resolvers = {
|
|
Query: {
|
|
rands: () => rands,
|
|
rand: (_, { id }) => rands.find(rand => rand.id === id),
|
|
},
|
|
};
|
|
|
|
const schema = makeExecutableSchema({
|
|
typeDefs,
|
|
resolvers,
|
|
});
|
|
|
|
module.exports = function run(context, request) {
|
|
graphqlAzureFunctions({ schema })(context, request);
|
|
};
|
|
```
|
|
|
|
### GraphiQL
|
|
|
|
```javascript
|
|
const { graphiqlAzureFunctions } = require('apollo-server-azure-functions');
|
|
|
|
export function run(context, request) {
|
|
let query = `
|
|
{
|
|
rands {
|
|
id
|
|
rand
|
|
}
|
|
}
|
|
`;
|
|
|
|
// End point points to the path to the GraphQL API function
|
|
graphiqlAzureFunctions({ endpointURL: '/api/graphql', query })(
|
|
context,
|
|
request,
|
|
);
|
|
}
|
|
```
|