mirror of
https://github.com/vale981/tridactyl
synced 2025-03-05 09:31:41 -05:00
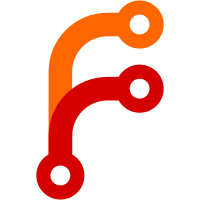
This rule requires adding a new set of rules, tslint-etc. no-unused-declaration used to be available in tslint:recommended but was deprecated when --noUnusedVariables was added to typescript. The problem with using TypeScript's --noUnusedVariables is that it turns unused declarations into an error and prevents compilation, which isn't fun when you're just prototyping things.
33 lines
787 B
TypeScript
33 lines
787 B
TypeScript
|
|
import { SymbolMetadata } from "./SymbolMetadata"
|
|
|
|
export class ClassMetadata {
|
|
constructor(
|
|
public members: Map<string, SymbolMetadata> = new Map<
|
|
string,
|
|
SymbolMetadata
|
|
>(),
|
|
) {}
|
|
|
|
public setMember(name: string, s: SymbolMetadata) {
|
|
this.members.set(name, s)
|
|
}
|
|
|
|
public getMember(name: string) {
|
|
return this.members.get(name)
|
|
}
|
|
|
|
public getMembers() {
|
|
return this.members.keys()
|
|
}
|
|
|
|
public toConstructor() {
|
|
return (
|
|
`new ClassMetadata(new Map<string, SymbolMetadata>([` +
|
|
Array.from(this.members.entries())
|
|
.map(([n, m]) => `[${JSON.stringify(n)}, ${m.toConstructor()}]`)
|
|
.join(",\n") +
|
|
`]))`
|
|
)
|
|
}
|
|
}
|