mirror of
https://github.com/vale981/ray
synced 2025-03-05 18:11:42 -05:00
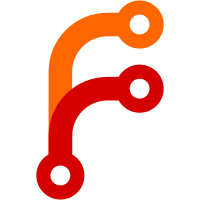
Clean up the ci/ directory. This means getting rid of the travis/ path completely and moving the files into sensible subdirectories. Details: - Moves everything under ci/travis into subdirectories, e.g. ci/build, ci/lint, etc. - Minor adjustments to some scripts (variable renames) - Removes the outdated (unused) asan tests
35 lines
962 B
Python
35 lines
962 B
Python
import argparse
|
|
import os
|
|
import shutil
|
|
import subprocess
|
|
import tempfile
|
|
|
|
|
|
def build_multinode_image(source_image: str, target_image: str):
|
|
"""Build docker image from source_image.
|
|
|
|
This docker image will contain packages needed for the fake multinode
|
|
docker cluster to work.
|
|
"""
|
|
tempdir = tempfile.mkdtemp()
|
|
|
|
dockerfile = os.path.join(tempdir, "Dockerfile")
|
|
with open(dockerfile, "wt") as f:
|
|
f.write(f"FROM {source_image}\n")
|
|
f.write("RUN sudo apt update\n")
|
|
f.write("RUN sudo apt install -y openssh-server\n")
|
|
|
|
subprocess.check_output(
|
|
f"docker build -t {target_image} .", shell=True, cwd=tempdir
|
|
)
|
|
|
|
shutil.rmtree(tempdir)
|
|
|
|
|
|
if __name__ == "__main__":
|
|
parser = argparse.ArgumentParser()
|
|
parser.add_argument("source_image", type=str)
|
|
parser.add_argument("target_image", type=str)
|
|
args = parser.parse_args()
|
|
|
|
build_multinode_image(args.source_image, args.target_image)
|