mirror of
https://github.com/vale981/ray
synced 2025-03-13 14:46:38 -04:00
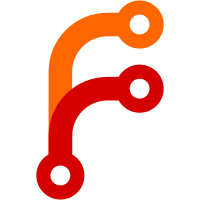
* Rename object_id -> ObjectID. * Rename ray_logger -> RayLogger. * rename task_id -> TaskID, actor_id -> ActorID, function_id -> FunctionID * Rename plasma_store_info -> PlasmaStoreInfo. * Rename plasma_store_state -> PlasmaStoreState. * Rename plasma_object -> PlasmaObject. * Rename object_request -> ObjectRequests. * Rename eviction_state -> EvictionState. * Bug fix. * rename db_handle -> DBHandle * Rename local_scheduler_state -> LocalSchedulerState. * rename db_client_id -> DBClientID * rename task -> Task * make redis.c C++ compatible * Rename scheduling_algorithm_state -> SchedulingAlgorithmState. * Rename plasma_connection -> PlasmaConnection. * Rename client_connection -> ClientConnection. * Fixes from rebase. * Rename local_scheduler_client -> LocalSchedulerClient. * Rename object_buffer -> ObjectBuffer. * Rename client -> Client. * Rename notification_queue -> NotificationQueue. * Rename object_get_requests -> ObjectGetRequests. * Rename get_request -> GetRequest. * Rename object_info -> ObjectInfo. * Rename scheduler_object_info -> SchedulerObjectInfo. * Rename local_scheduler -> LocalScheduler and some fixes. * Rename local_scheduler_info -> LocalSchedulerInfo. * Rename global_scheduler_state -> GlobalSchedulerState. * Rename global_scheduler_policy_state -> GlobalSchedulerPolicyState. * Rename object_size_entry -> ObjectSizeEntry. * Rename aux_address_entry -> AuxAddressEntry. * Rename various ID helper methods. * Rename Task helper methods. * Rename db_client_cache_entry -> DBClientCacheEntry. * Rename local_actor_info -> LocalActorInfo. * Rename actor_info -> ActorInfo. * Rename retry_info -> RetryInfo. * Rename actor_notification_table_subscribe_data -> ActorNotificationTableSubscribeData. * Rename local_scheduler_table_send_info_data -> LocalSchedulerTableSendInfoData. * Rename table_callback_data -> TableCallbackData. * Rename object_info_subscribe_data -> ObjectInfoSubscribeData. * Rename local_scheduler_table_subscribe_data -> LocalSchedulerTableSubscribeData. * Rename more redis call data structures. * Rename photon_conn PhotonConnection. * Rename photon_mock -> PhotonMock. * Fix formatting errors.
175 lines
6.2 KiB
C
175 lines
6.2 KiB
C
#ifndef TABLE_H
|
|
#define TABLE_H
|
|
|
|
#include "uthash.h"
|
|
#include "stdbool.h"
|
|
|
|
#include "common.h"
|
|
#include "db.h"
|
|
|
|
typedef struct TableCallbackData TableCallbackData;
|
|
|
|
typedef void *table_done_callback;
|
|
|
|
/* The callback called when the database operation hasn't completed after
|
|
* the number of retries specified for the operation.
|
|
*
|
|
* @param id The unique ID that identifies this callback. Examples include an
|
|
* object ID or task ID.
|
|
* @param user_context The state context for the callback. This is equivalent
|
|
* to the user_context field in TableCallbackData.
|
|
* @param user_data A data argument for the callback. This is equivalent to the
|
|
* data field in TableCallbackData. The user is responsible for
|
|
* freeing user_data.
|
|
*/
|
|
typedef void (*table_fail_callback)(UniqueID id,
|
|
void *user_context,
|
|
void *user_data);
|
|
|
|
typedef void (*table_retry_callback)(TableCallbackData *callback_data);
|
|
|
|
/**
|
|
* Data structure consolidating the retry related variables. If a NULL
|
|
* RetryInfo struct is used, the default behavior will be to retry infinitely
|
|
* many times.
|
|
*/
|
|
typedef struct {
|
|
/** Number of retries. This field will be decremented every time a retry
|
|
* occurs (unless the value is -1). If this value is -1, then there will be
|
|
* infinitely many retries. */
|
|
int num_retries;
|
|
/** Timeout, in milliseconds. */
|
|
uint64_t timeout;
|
|
/** The callback that will be called if there are no more retries left. */
|
|
table_fail_callback fail_callback;
|
|
} RetryInfo;
|
|
|
|
struct TableCallbackData {
|
|
/** ID of the entry in the table that we are going to look up, remove or add.
|
|
*/
|
|
UniqueID id;
|
|
/** A label to identify the original request for logging purposes. */
|
|
const char *label;
|
|
/** The callback that will be called when results is returned. */
|
|
table_done_callback done_callback;
|
|
/** The callback that will be called to initiate the next try. */
|
|
table_retry_callback retry_callback;
|
|
/** Retry information containing the remaining number of retries, the timeout
|
|
* before the next retry, and a pointer to the failure callback.
|
|
*/
|
|
RetryInfo retry;
|
|
/** Pointer to the data that is entered into the table. This can be used to
|
|
* pass the result of the call to the callback. The callback takes ownership
|
|
* over this data and will free it. */
|
|
void *data;
|
|
/** Pointer to the data used internally to handle multiple database requests.
|
|
*/
|
|
void *requests_info;
|
|
/** User context. */
|
|
void *user_context;
|
|
/** Handle to db. */
|
|
DBHandle *db_handle;
|
|
/** Handle to timer. */
|
|
int64_t timer_id;
|
|
UT_hash_handle hh; /* makes this structure hashable */
|
|
};
|
|
|
|
/**
|
|
* Function to handle the timeout event.
|
|
*
|
|
* @param loop Event loop.
|
|
* @param timer_id Timer identifier.
|
|
* @param context Pointer to the callback data for the object table
|
|
* @return Timeout to reset the timer if we need to try again, or
|
|
* EVENT_LOOP_TIMER_DONE if retry_count == 0.
|
|
*/
|
|
int64_t table_timeout_handler(event_loop *loop,
|
|
int64_t timer_id,
|
|
void *context);
|
|
|
|
/**
|
|
* Initialize the table callback and call the retry_callback for the first time.
|
|
*
|
|
* @param db_handle Database handle.
|
|
* @param id ID of the object that is looked up, added or removed.
|
|
* @param label A string label to identify the type of table request for
|
|
* logging purposes.
|
|
* @param data Data entered into the table. Shall be freed by the user.
|
|
* @param retry Retry relevant information: retry timeout, number of remaining
|
|
* retries, and retry callback.
|
|
* @param done_callback Function to be called when database returns result.
|
|
* @param fail_callback Function to be called when number of retries is
|
|
* exhausted.
|
|
* @param user_context Context that can be provided by the user and will be
|
|
* passed on to the various callbacks.
|
|
* @return New table callback data struct.
|
|
*/
|
|
TableCallbackData *init_table_callback(DBHandle *db_handle,
|
|
UniqueID id,
|
|
const char *label,
|
|
OWNER void *data,
|
|
RetryInfo *retry,
|
|
table_done_callback done_callback,
|
|
table_retry_callback retry_callback,
|
|
void *user_context);
|
|
|
|
/**
|
|
* Destroy any state associated with the callback data. This removes all
|
|
* associated state from the outstanding callbacks hash table and frees any
|
|
* associated memory. This does not remove any associated timer events.
|
|
*
|
|
* @param callback_data The pointer to the data structure of the callback we
|
|
* want to remove.
|
|
* @return Void.
|
|
*/
|
|
void destroy_table_callback(TableCallbackData *callback_data);
|
|
|
|
/**
|
|
* Destroy all state events associated with the callback data, including memory
|
|
* and timer events.
|
|
*
|
|
* @param callback_data The pointer to the data structure of the callback we
|
|
* want to remove.
|
|
* @return Void.
|
|
*/
|
|
void destroy_timer_callback(event_loop *loop, TableCallbackData *callback_data);
|
|
|
|
/**
|
|
* Add an outstanding callback entry.
|
|
*
|
|
* @param callback_data The pointer to the data structure of the callback we
|
|
* want to insert.
|
|
* @return None.
|
|
*/
|
|
void outstanding_callbacks_add(TableCallbackData *callback_data);
|
|
|
|
/**
|
|
* Find an outstanding callback entry.
|
|
*
|
|
* @param key The key for the outstanding callbacks hash table. We use the
|
|
* timer ID assigned by the Redis ae event loop.
|
|
* @return Returns the callback data if found, NULL otherwise.
|
|
*/
|
|
TableCallbackData *outstanding_callbacks_find(int64_t key);
|
|
|
|
/**
|
|
* Remove an outstanding callback entry. This only removes the callback entry
|
|
* from the hash table. It does not free the entry or remove any associated
|
|
* timer events.
|
|
*
|
|
* @param callback_data The pointer to the data structure of the callback we
|
|
* want to remove.
|
|
* @return Void.
|
|
*/
|
|
void outstanding_callbacks_remove(TableCallbackData *callback_data);
|
|
|
|
/**
|
|
* Destroy all outstanding callbacks and remove their associated timer events
|
|
* from the event loop.
|
|
*
|
|
* @param loop The event loop from which we want to remove the timer events.
|
|
* @return Void.
|
|
*/
|
|
void destroy_outstanding_callbacks(event_loop *loop);
|
|
|
|
#endif /* TABLE_H */
|