mirror of
https://github.com/vale981/ray
synced 2025-03-05 18:11:42 -05:00
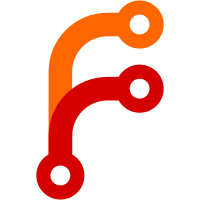
Adds a unit-tested and restructured ray_release package for running release tests. Relevant changes in behavior: Per default, Buildkite will wait for the wheels of the current commit to be available. Alternatively, users can a) specify a different commit hash, b) a wheels URL (which we will also wait for to be available) or c) specify a branch (or user/branch combination), in which case the latest available wheels will be used (e.g. if master is passed, behavior matches old default behavior). The main subpackages are: Cluster manager: Creates cluster envs/computes, starts cluster, terminates cluster Command runner: Runs commands, e.g. as client command or sdk command File manager: Uploads/downloads files to/from session Reporter: Reports results (e.g. to database) Much of the code base is unit tested, but there are probably some pieces missing. Example build (waited for wheels to be built): https://buildkite.com/ray-project/kf-dev/builds/51#_ Wheel build: https://buildkite.com/ray-project/ray-builders-branch/builds/6023
51 lines
1.3 KiB
Python
51 lines
1.3 KiB
Python
from typing import Optional
|
|
|
|
from anyscale.sdk.anyscale_client.sdk import AnyscaleSDK
|
|
|
|
from ray_release.logger import logger
|
|
from ray_release.util import get_anyscale_sdk
|
|
|
|
|
|
LAST_LOGS_LENGTH = 10
|
|
|
|
|
|
def find_cloud_by_name(
|
|
cloud_name: str, sdk: Optional[AnyscaleSDK] = None
|
|
) -> Optional[str]:
|
|
sdk = sdk or get_anyscale_sdk()
|
|
|
|
cloud_id = None
|
|
logger.info(f"Looking up cloud with name `{cloud_name}`. ")
|
|
|
|
paging_token = None
|
|
while not cloud_id:
|
|
result = sdk.search_clouds(
|
|
clouds_query=dict(paging=dict(count=50, paging_token=paging_token))
|
|
)
|
|
|
|
paging_token = result.metadata.next_paging_token
|
|
|
|
for res in result.results:
|
|
if res.name == cloud_name:
|
|
cloud_id = res.id
|
|
logger.info(f"Found cloud with name `{cloud_name}` as `{cloud_id}`")
|
|
break
|
|
|
|
if not paging_token or cloud_id or not len(result.results):
|
|
break
|
|
|
|
return cloud_id
|
|
|
|
|
|
def get_project_name(project_id: str, sdk: Optional[AnyscaleSDK] = None) -> str:
|
|
sdk = sdk or get_anyscale_sdk()
|
|
|
|
result = sdk.get_project(project_id)
|
|
return result.result.name
|
|
|
|
|
|
def get_cluster_name(cluster_id: str, sdk: Optional[AnyscaleSDK] = None) -> str:
|
|
sdk = sdk or get_anyscale_sdk()
|
|
|
|
result = sdk.get_cluster(cluster_id)
|
|
return result.result.name
|