mirror of
https://github.com/vale981/ray
synced 2025-03-06 18:41:40 -05:00
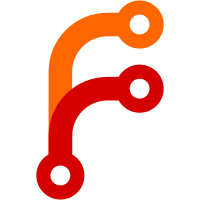
Removes unnecessary pandas usage from AIR examples. Helps ensure users do not follow bad practices.
523 lines
55 KiB
Text
523 lines
55 KiB
Text
{
|
|
"cells": [
|
|
{
|
|
"cell_type": "markdown",
|
|
"id": "0d385409",
|
|
"metadata": {},
|
|
"source": [
|
|
"# Training a model with distributed LightGBM\n",
|
|
"In this example we will train a model in Ray AIR using distributed LightGBM."
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "markdown",
|
|
"id": "07d92cee",
|
|
"metadata": {},
|
|
"source": [
|
|
"Let's start with installing our dependencies:"
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "code",
|
|
"execution_count": 1,
|
|
"id": "86131abe",
|
|
"metadata": {},
|
|
"outputs": [],
|
|
"source": [
|
|
"!pip install -qU \"ray[tune]\" lightgbm_ray"
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "markdown",
|
|
"id": "135fc884",
|
|
"metadata": {},
|
|
"source": [
|
|
"Then we need some imports:"
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "code",
|
|
"execution_count": 4,
|
|
"id": "102ef1ac",
|
|
"metadata": {},
|
|
"outputs": [],
|
|
"source": [
|
|
"from typing import Tuple\n",
|
|
"\n",
|
|
"import ray\n",
|
|
"from ray.train.batch_predictor import BatchPredictor\n",
|
|
"from ray.train.lightgbm import LightGBMPredictor\n",
|
|
"from ray.data.preprocessors.chain import Chain\n",
|
|
"from ray.data.preprocessors.encoder import Categorizer\n",
|
|
"from ray.train.lightgbm import LightGBMTrainer\n",
|
|
"from ray.data.dataset import Dataset\n",
|
|
"from ray.air.result import Result\n",
|
|
"from ray.air.util.datasets import train_test_split\n",
|
|
"from ray.data.preprocessors import StandardScaler"
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "markdown",
|
|
"id": "c7d102bd",
|
|
"metadata": {},
|
|
"source": [
|
|
"Next we define a function to load our train, validation, and test datasets."
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "code",
|
|
"execution_count": 13,
|
|
"id": "f1f35cd7",
|
|
"metadata": {},
|
|
"outputs": [],
|
|
"source": [
|
|
"def prepare_data() -> Tuple[Dataset, Dataset, Dataset]:\n",
|
|
" dataset = ray.data.read_csv(\"s3://air-example-data/breast_cancer_with_categorical.csv\")\n",
|
|
" train_dataset, valid_dataset = train_test_split(dataset, test_size=0.3)\n",
|
|
" test_dataset = valid_dataset.map_batches(lambda df: df.drop(\"target\", axis=1), batch_format=\"pandas\")\n",
|
|
" return train_dataset, valid_dataset, test_dataset"
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "markdown",
|
|
"id": "8f7afbce",
|
|
"metadata": {},
|
|
"source": [
|
|
"The following function will create a LightGBM trainer, train it, and return the result."
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "code",
|
|
"execution_count": 14,
|
|
"id": "fefcbc8a",
|
|
"metadata": {},
|
|
"outputs": [],
|
|
"source": [
|
|
"def train_lightgbm(num_workers: int, use_gpu: bool = False) -> Result:\n",
|
|
" train_dataset, valid_dataset, _ = prepare_data()\n",
|
|
"\n",
|
|
" # Scale some random columns, and categorify the categorical_column,\n",
|
|
" # allowing LightGBM to use its built-in categorical feature support\n",
|
|
" columns_to_scale = [\"mean radius\", \"mean texture\"]\n",
|
|
" preprocessor = Chain(\n",
|
|
" Categorizer([\"categorical_column\"]), StandardScaler(columns=columns_to_scale)\n",
|
|
" )\n",
|
|
"\n",
|
|
" # LightGBM specific params\n",
|
|
" params = {\n",
|
|
" \"objective\": \"binary\",\n",
|
|
" \"metric\": [\"binary_logloss\", \"binary_error\"],\n",
|
|
" }\n",
|
|
"\n",
|
|
" trainer = LightGBMTrainer(\n",
|
|
" scaling_config={\n",
|
|
" \"num_workers\": num_workers,\n",
|
|
" \"use_gpu\": use_gpu,\n",
|
|
" },\n",
|
|
" label_column=\"target\",\n",
|
|
" params=params,\n",
|
|
" datasets={\"train\": train_dataset, \"valid\": valid_dataset},\n",
|
|
" preprocessor=preprocessor,\n",
|
|
" num_boost_round=100,\n",
|
|
" )\n",
|
|
" result = trainer.fit()\n",
|
|
" print(result.metrics)\n",
|
|
"\n",
|
|
" return result"
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "markdown",
|
|
"id": "04d278ae",
|
|
"metadata": {},
|
|
"source": [
|
|
"Once we have the result, we can do batch inference on the obtained model. Let's define a utility function for this."
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "code",
|
|
"execution_count": 15,
|
|
"id": "3f1d0c19",
|
|
"metadata": {},
|
|
"outputs": [],
|
|
"source": [
|
|
"def predict_lightgbm(result: Result):\n",
|
|
" _, _, test_dataset = prepare_data()\n",
|
|
" batch_predictor = BatchPredictor.from_checkpoint(\n",
|
|
" result.checkpoint, LightGBMPredictor\n",
|
|
" )\n",
|
|
"\n",
|
|
" predicted_labels = (\n",
|
|
" batch_predictor.predict(test_dataset)\n",
|
|
" .map_batches(lambda df: (df > 0.5).astype(int), batch_format=\"pandas\")\n",
|
|
" )\n",
|
|
" print(f\"PREDICTED LABELS\")\n",
|
|
" predicted_labels.show()\n",
|
|
"\n",
|
|
" shap_values = batch_predictor.predict(test_dataset, pred_contrib=True)\n",
|
|
" print(f\"SHAP VALUES\")\n",
|
|
" shap_values.show()"
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "markdown",
|
|
"id": "2bb0e5df",
|
|
"metadata": {},
|
|
"source": [
|
|
"Now we can run the training:"
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "code",
|
|
"execution_count": 16,
|
|
"id": "8244ff3c",
|
|
"metadata": {},
|
|
"outputs": [
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"2022-06-22 17:26:41,346\tWARNING read_api.py:260 -- The number of blocks in this dataset (1) limits its parallelism to 1 concurrent tasks. This is much less than the number of available CPU slots in the cluster. Use `.repartition(n)` to increase the number of dataset blocks.\n",
|
|
"Map_Batches: 100%|██████████| 1/1 [00:00<00:00, 46.26it/s]\n"
|
|
]
|
|
},
|
|
{
|
|
"data": {
|
|
"text/html": [
|
|
"== Status ==<br>Current time: 2022-06-22 17:26:56 (running for 00:00:14.07)<br>Memory usage on this node: 10.0/31.0 GiB<br>Using FIFO scheduling algorithm.<br>Resources requested: 0/8 CPUs, 0/0 GPUs, 0.0/13.32 GiB heap, 0.0/6.66 GiB objects<br>Result logdir: /home/ubuntu/ray_results/LightGBMTrainer_2022-06-22_17-26-41<br>Number of trials: 1/1 (1 TERMINATED)<br><table>\n",
|
|
"<thead>\n",
|
|
"<tr><th>Trial name </th><th>status </th><th>loc </th><th style=\"text-align: right;\"> iter</th><th style=\"text-align: right;\"> total time (s)</th><th style=\"text-align: right;\"> train-binary_logloss</th><th style=\"text-align: right;\"> train-binary_error</th><th style=\"text-align: right;\"> valid-binary_logloss</th></tr>\n",
|
|
"</thead>\n",
|
|
"<tbody>\n",
|
|
"<tr><td>LightGBMTrainer_7b049_00000</td><td>TERMINATED</td><td>172.31.43.110:1491578</td><td style=\"text-align: right;\"> 100</td><td style=\"text-align: right;\"> 10.9726</td><td style=\"text-align: right;\"> 0.000574522</td><td style=\"text-align: right;\"> 0</td><td style=\"text-align: right;\"> 0.171898</td></tr>\n",
|
|
"</tbody>\n",
|
|
"</table><br><br>"
|
|
],
|
|
"text/plain": [
|
|
"<IPython.core.display.HTML object>"
|
|
]
|
|
},
|
|
"metadata": {},
|
|
"output_type": "display_data"
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"UserWarning: cpus_per_actor is set to less than 2. Distributed LightGBM needs at least 2 CPUs per actor to train efficiently. This may lead to a degradation of performance during training.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491578)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491578)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1491578)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Float64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491578)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1491578)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.UInt64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491578)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1491578)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/xgboost/compat.py:31: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491578)\u001b[0m from pandas import MultiIndex, Int64Index\n",
|
|
"\u001b[2m\u001b[36m(LightGBMTrainer pid=1491578)\u001b[0m UserWarning: Dataset 'train' has 1 blocks, which is less than the `num_workers` 2. This dataset will be automatically repartitioned to 2 blocks.\n",
|
|
"\u001b[2m\u001b[36m(LightGBMTrainer pid=1491578)\u001b[0m UserWarning: Dataset 'valid' has 1 blocks, which is less than the `num_workers` 2. This dataset will be automatically repartitioned to 2 blocks.\n",
|
|
"\u001b[2m\u001b[36m(LightGBMTrainer pid=1491578)\u001b[0m UserWarning: cpus_per_actor is set to less than 2. Distributed LightGBM needs at least 2 CPUs per actor to train efficiently. This may lead to a degradation of performance during training.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491651)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/xgboost/compat.py:31: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491651)\u001b[0m from pandas import MultiIndex, Int64Index\n",
|
|
"\u001b[2m\u001b[36m(pid=1491651)\u001b[0m FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491651)\u001b[0m FutureWarning: pandas.Float64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491651)\u001b[0m FutureWarning: pandas.UInt64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491653)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491653)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1491653)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Float64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491653)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1491653)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.UInt64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491653)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1491653)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/xgboost/compat.py:31: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491653)\u001b[0m from pandas import MultiIndex, Int64Index\n",
|
|
"\u001b[2m\u001b[36m(pid=1491652)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491652)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1491652)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Float64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491652)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1491652)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.UInt64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491652)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1491652)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/xgboost/compat.py:31: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491652)\u001b[0m from pandas import MultiIndex, Int64Index\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491653)\u001b[0m 2022-06-22 17:26:50,509\tWARNING __init__.py:190 -- DeprecationWarning: `ray.worker.get_resource_ids` is a private attribute and access will be removed in a future Ray version.\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491652)\u001b[0m 2022-06-22 17:26:50,658\tWARNING __init__.py:190 -- DeprecationWarning: `ray.worker.get_resource_ids` is a private attribute and access will be removed in a future Ray version.\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491653)\u001b[0m [LightGBM] [Info] Trying to bind port 59039...\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491653)\u001b[0m [LightGBM] [Info] Binding port 59039 succeeded\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491653)\u001b[0m [LightGBM] [Info] Listening...\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491652)\u001b[0m [LightGBM] [Info] Trying to bind port 46955...\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491652)\u001b[0m [LightGBM] [Info] Binding port 46955 succeeded\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491652)\u001b[0m [LightGBM] [Info] Listening...\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491652)\u001b[0m [LightGBM] [Warning] Connecting to rank 1 failed, waiting for 200 milliseconds\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491653)\u001b[0m UserWarning: Overriding the parameters from Reference Dataset.\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491653)\u001b[0m UserWarning: categorical_column in param dict is overridden.\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491652)\u001b[0m UserWarning: Overriding the parameters from Reference Dataset.\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491652)\u001b[0m UserWarning: categorical_column in param dict is overridden.\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491653)\u001b[0m [LightGBM] [Info] Connected to rank 0\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491653)\u001b[0m [LightGBM] [Info] Local rank: 1, total number of machines: 2\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491653)\u001b[0m [LightGBM] [Warning] num_threads is set=1, n_jobs=-1 will be ignored. Current value: num_threads=1\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491652)\u001b[0m [LightGBM] [Info] Connected to rank 1\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491652)\u001b[0m [LightGBM] [Info] Local rank: 0, total number of machines: 2\n",
|
|
"\u001b[2m\u001b[36m(_RemoteRayLightGBMActor pid=1491652)\u001b[0m [LightGBM] [Warning] num_threads is set=1, n_jobs=-1 will be ignored. Current value: num_threads=1\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"\u001b[2m\u001b[36m(_QueueActor pid=1491650)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(_QueueActor pid=1491650)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(_QueueActor pid=1491650)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Float64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(_QueueActor pid=1491650)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(_QueueActor pid=1491650)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.UInt64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(_QueueActor pid=1491650)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(_QueueActor pid=1491650)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/xgboost/compat.py:31: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(_QueueActor pid=1491650)\u001b[0m from pandas import MultiIndex, Int64Index\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"Result for LightGBMTrainer_7b049_00000:\n",
|
|
" date: 2022-06-22_17-26-53\n",
|
|
" done: false\n",
|
|
" experiment_id: b4a87c26a7604a43baf895755d4f16b3\n",
|
|
" hostname: ip-172-31-43-110\n",
|
|
" iterations_since_restore: 1\n",
|
|
" node_ip: 172.31.43.110\n",
|
|
" pid: 1491578\n",
|
|
" should_checkpoint: true\n",
|
|
" time_since_restore: 8.369545459747314\n",
|
|
" time_this_iter_s: 8.369545459747314\n",
|
|
" time_total_s: 8.369545459747314\n",
|
|
" timestamp: 1655918813\n",
|
|
" timesteps_since_restore: 0\n",
|
|
" train-binary_error: 0.5175879396984925\n",
|
|
" train-binary_logloss: 0.6302848981539763\n",
|
|
" training_iteration: 1\n",
|
|
" trial_id: 7b049_00000\n",
|
|
" valid-binary_error: 0.2\n",
|
|
" valid-binary_logloss: 0.558752017793943\n",
|
|
" warmup_time: 0.008721590042114258\n",
|
|
" \n",
|
|
"Result for LightGBMTrainer_7b049_00000:\n",
|
|
" date: 2022-06-22_17-26-56\n",
|
|
" done: true\n",
|
|
" experiment_id: b4a87c26a7604a43baf895755d4f16b3\n",
|
|
" experiment_tag: '0'\n",
|
|
" hostname: ip-172-31-43-110\n",
|
|
" iterations_since_restore: 100\n",
|
|
" node_ip: 172.31.43.110\n",
|
|
" pid: 1491578\n",
|
|
" should_checkpoint: true\n",
|
|
" time_since_restore: 10.972588300704956\n",
|
|
" time_this_iter_s: 0.027977466583251953\n",
|
|
" time_total_s: 10.972588300704956\n",
|
|
" timestamp: 1655918816\n",
|
|
" timesteps_since_restore: 0\n",
|
|
" train-binary_error: 0.0\n",
|
|
" train-binary_logloss: 0.0005745220956391456\n",
|
|
" training_iteration: 100\n",
|
|
" trial_id: 7b049_00000\n",
|
|
" valid-binary_error: 0.058823529411764705\n",
|
|
" valid-binary_logloss: 0.17189847605331432\n",
|
|
" warmup_time: 0.008721590042114258\n",
|
|
" \n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"2022-06-22 17:26:56,406\tINFO tune.py:734 -- Total run time: 14.73 seconds (14.06 seconds for the tuning loop).\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"{'train-binary_logloss': 0.0005745220956391456, 'train-binary_error': 0.0, 'valid-binary_logloss': 0.17189847605331432, 'valid-binary_error': 0.058823529411764705, 'time_this_iter_s': 0.027977466583251953, 'should_checkpoint': True, 'done': True, 'timesteps_total': None, 'episodes_total': None, 'training_iteration': 100, 'trial_id': '7b049_00000', 'experiment_id': 'b4a87c26a7604a43baf895755d4f16b3', 'date': '2022-06-22_17-26-56', 'timestamp': 1655918816, 'time_total_s': 10.972588300704956, 'pid': 1491578, 'hostname': 'ip-172-31-43-110', 'node_ip': '172.31.43.110', 'config': {}, 'time_since_restore': 10.972588300704956, 'timesteps_since_restore': 0, 'iterations_since_restore': 100, 'warmup_time': 0.008721590042114258, 'experiment_tag': '0'}\n"
|
|
]
|
|
}
|
|
],
|
|
"source": [
|
|
"result = train_lightgbm(num_workers=2, use_gpu=False)"
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "markdown",
|
|
"id": "d7155d9b",
|
|
"metadata": {},
|
|
"source": [
|
|
"And perform inference on the obtained model:"
|
|
]
|
|
},
|
|
{
|
|
"cell_type": "code",
|
|
"execution_count": 17,
|
|
"id": "871c9be6",
|
|
"metadata": {},
|
|
"outputs": [
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"2022-06-22 17:26:57,517\tWARNING read_api.py:260 -- The number of blocks in this dataset (1) limits its parallelism to 1 concurrent tasks. This is much less than the number of available CPU slots in the cluster. Use `.repartition(n)` to increase the number of dataset blocks.\n",
|
|
"Map_Batches: 100%|██████████| 1/1 [00:00<00:00, 50.96it/s]\n",
|
|
"Map_Batches: 0%| | 0/1 [00:00<?, ?it/s]\u001b[2m\u001b[36m(pid=1491998)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491998)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1491998)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Float64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491998)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1491998)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.UInt64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491998)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1491998)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/xgboost/compat.py:31: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1491998)\u001b[0m from pandas import MultiIndex, Int64Index\n",
|
|
"Map Progress (1 actors 1 pending): 100%|██████████| 1/1 [00:02<00:00, 2.05s/it]\n",
|
|
"Map_Batches: 100%|██████████| 1/1 [00:00<00:00, 75.07it/s]\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"PREDICTED LABELS\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 0}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 0}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 0}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 1}\n",
|
|
"{'predictions': 0}\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"Map_Batches: 0%| | 0/1 [00:00<?, ?it/s]\u001b[2m\u001b[36m(pid=1492031)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1492031)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1492031)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Float64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1492031)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1492031)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.UInt64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1492031)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1492031)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/xgboost/compat.py:31: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1492031)\u001b[0m from pandas import MultiIndex, Int64Index\n",
|
|
"\u001b[2m\u001b[36m(pid=1492033)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1492033)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1492033)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Float64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1492033)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1492033)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.UInt64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1492033)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1492033)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/xgboost/compat.py:31: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1492033)\u001b[0m from pandas import MultiIndex, Int64Index\n",
|
|
"Map Progress (1 actors 1 pending): 100%|██████████| 1/1 [00:02<00:00, 2.09s/it]\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"SHAP VALUES\n",
|
|
"{'predictions_0': 0.006121974664714535, 'predictions_1': 0.8940294162424869, 'predictions_2': -0.013623909529011522, 'predictions_3': -0.26580572803883, 'predictions_4': 0.2897686828261492, 'predictions_5': -0.03784232120648852, 'predictions_6': 0.021865334852359534, 'predictions_7': 1.1753326094382734, 'predictions_8': -0.02525466292349231, 'predictions_9': 0.0733463992354119, 'predictions_10': 0.09191922035401615, 'predictions_11': -0.0035196096494634313, 'predictions_12': 0.20211476104388482, 'predictions_13': 0.7813488658944929, 'predictions_14': 0.10000464816891827, 'predictions_15': 0.11543593649642907, 'predictions_16': -0.009732477634862284, 'predictions_17': 0.19117650484758314, 'predictions_18': -0.17600075102817322, 'predictions_19': 0.5829434737180024, 'predictions_20': 1.4220773445509465, 'predictions_21': 0.6086211783805069, 'predictions_22': 2.0031654232526925, 'predictions_23': 0.3090376110779834, 'predictions_24': -0.21156467772251453, 'predictions_25': 0.14122943819731193, 'predictions_26': -0.1324700025487787, 'predictions_27': 0.8280650504246968, 'predictions_28': 0.03147457104755769, 'predictions_29': 0.00029604737237433516, 'predictions_30': 0.024336487839325866, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.00565090216762466, 'predictions_1': 0.7173247917145018, 'predictions_2': -0.01352989648419376, 'predictions_3': -0.204508963539279, 'predictions_4': -0.11703564338083555, 'predictions_5': 0.059858710083059874, 'predictions_6': 0.06974454296095976, 'predictions_7': 1.5952991804773315, 'predictions_8': 0.30494490847895245, 'predictions_9': 0.03770331660034111, 'predictions_10': 0.08779844216179675, 'predictions_11': 0.0001818669974550241, 'predictions_12': -0.10871732001356472, 'predictions_13': 0.49872871949407244, 'predictions_14': 0.16083030838859202, 'predictions_15': 0.4071487385487001, 'predictions_16': -0.00920287075428388, 'predictions_17': 0.21519060265555054, 'predictions_18': -0.24141319659570365, 'predictions_19': -0.19394859165532527, 'predictions_20': 1.2358452648954865, 'predictions_21': 0.16127531717942642, 'predictions_22': 1.3397755121893355, 'predictions_23': 0.24271016133964965, 'predictions_24': -0.11296858156987878, 'predictions_25': 0.21775788278030012, 'predictions_26': 0.8594002204044787, 'predictions_27': 1.0571631081079365, 'predictions_28': 0.06338809094380635, 'predictions_29': 0.14952090064808415, 'predictions_30': -0.020191656254497082, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.0011410972769028797, 'predictions_1': -0.023112580054428615, 'predictions_2': 0.0015007474035067395, 'predictions_3': -0.3960490192373774, 'predictions_4': -0.30646108596137317, 'predictions_5': -0.015606280874156383, 'predictions_6': -0.10875176916234583, 'predictions_7': -2.3253286264519457, 'predictions_8': 0.2758843675860649, 'predictions_9': 0.029091310311824298, 'predictions_10': -0.057950348636255644, 'predictions_11': -0.00017555498393944432, 'predictions_12': -0.4136454204716676, 'predictions_13': -0.3629735922139978, 'predictions_14': 0.04232756741319012, 'predictions_15': 0.06936920198392876, 'predictions_16': 0.010307144611165166, 'predictions_17': -0.4063116213440989, 'predictions_18': -0.07826460708005233, 'predictions_19': 0.28668914680505037, 'predictions_20': -2.0034181076720015, 'predictions_21': -0.4289092806234529, 'predictions_22': -2.059807308089095, 'predictions_23': -0.2625534917898286, 'predictions_24': -1.0607560950436483, 'predictions_25': -0.13241418825219023, 'predictions_26': -0.46713657128877134, 'predictions_27': -2.0707325110237127, 'predictions_28': -0.0212343580603297, 'predictions_29': -0.11761200100287779, 'predictions_30': 0.03805635018946682, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.003501920356918757, 'predictions_1': 0.9649889446638613, 'predictions_2': -0.011627077584939034, 'predictions_3': -0.33201537640627937, 'predictions_4': 0.2626117060870051, 'predictions_5': -0.0420997182498785, 'predictions_6': 0.05656763216450521, 'predictions_7': 1.076092179662977, 'predictions_8': -0.1396182169782879, 'predictions_9': -0.09872952353947571, 'predictions_10': 0.04378766056466948, 'predictions_11': 0.002478996394296549, 'predictions_12': 0.25042183813566526, 'predictions_13': 0.8751692867530225, 'predictions_14': 0.18679133739736484, 'predictions_15': 0.046846715006450504, 'predictions_16': -0.009211815518670832, 'predictions_17': 0.22485983912144494, 'predictions_18': -0.2861737431801593, 'predictions_19': -0.2533929278067911, 'predictions_20': 1.316951719635302, 'predictions_21': 1.1964971086769494, 'predictions_22': 1.2740098717427248, 'predictions_23': 0.25042580055967084, 'predictions_24': -0.4015257176668039, 'predictions_25': 0.17935395324361414, 'predictions_26': 1.126933988937795, 'predictions_27': 0.8031626612897146, 'predictions_28': 0.0771850514731471, 'predictions_29': 0.03755423306624511, 'predictions_30': -0.016833253240925238, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': -0.0034560551402153003, 'predictions_1': 0.5230708630376469, 'predictions_2': -0.015562114219360572, 'predictions_3': -0.1196402194436373, 'predictions_4': 0.4106482044292619, 'predictions_5': 0.06220233147046589, 'predictions_6': 0.12716114707514065, 'predictions_7': 1.3356455912614509, 'predictions_8': 0.1447514882444872, 'predictions_9': 0.12370386736447751, 'predictions_10': 0.07410456355721864, 'predictions_11': 0.012016763274156357, 'predictions_12': -0.10513441936331262, 'predictions_13': 0.7484191363603289, 'predictions_14': 0.18707788149117566, 'predictions_15': 0.3327881147491029, 'predictions_16': -0.009219336794413353, 'predictions_17': -0.10065740008750416, 'predictions_18': 0.16625881614886867, 'predictions_19': 0.23084551369873454, 'predictions_20': 1.358717098613538, 'predictions_21': 0.19175435277095332, 'predictions_22': 1.3375643842995248, 'predictions_23': 0.2926283902278477, 'predictions_24': 0.1146310032943002, 'predictions_25': 0.23343399483643015, 'predictions_26': 0.6034462734909513, 'predictions_27': 0.9230214841058666, 'predictions_28': 0.029594344165258104, 'predictions_29': 0.04913153000099999, 'predictions_30': 0.02341707352913655, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': -0.005102561841927789, 'predictions_1': 1.0861119112102469, 'predictions_2': -0.0154828846564582, 'predictions_3': -0.3088905099091714, 'predictions_4': 0.05779026036152443, 'predictions_5': 0.047351932324116885, 'predictions_6': 0.0876371219806605, 'predictions_7': 1.1210466016114495, 'predictions_8': -0.1252369777517682, 'predictions_9': 0.04572512843104436, 'predictions_10': 0.09245771221086214, 'predictions_11': 0.007753500238910626, 'predictions_12': 0.2309698163766563, 'predictions_13': 0.9684988783771291, 'predictions_14': 0.024511467599608535, 'predictions_15': 0.18657179919131872, 'predictions_16': -0.009212652411079585, 'predictions_17': -0.13395842318946233, 'predictions_18': 0.152376407447391, 'predictions_19': -0.28554273302892486, 'predictions_20': 1.3994697511973517, 'predictions_21': 0.5784457048607689, 'predictions_22': 1.3325378201278, 'predictions_23': 0.30730022154186687, 'predictions_24': 0.017237427138293876, 'predictions_25': 0.19484371531419448, 'predictions_26': 1.0716212980249242, 'predictions_27': 0.7424857548065319, 'predictions_28': 0.030110335845485465, 'predictions_29': 0.08677604394991238, 'predictions_30': -0.018230914497616164, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.011026642414565658, 'predictions_1': 1.0433621693813095, 'predictions_2': -0.00702393810808943, 'predictions_3': -0.2962479861350653, 'predictions_4': 0.20838486132625483, 'predictions_5': -0.07568934141814487, 'predictions_6': 0.026798049998736986, 'predictions_7': 1.2233970557267948, 'predictions_8': -0.07215770822854156, 'predictions_9': 0.016138237086580777, 'predictions_10': 0.04908427317188252, 'predictions_11': -0.013274124641011575, 'predictions_12': -0.16059386568879297, 'predictions_13': 0.38386374312584454, 'predictions_14': -0.03476748264814593, 'predictions_15': -0.5225211720205649, 'predictions_16': -0.009220168600202043, 'predictions_17': -0.15278574495418593, 'predictions_18': 0.12911665421378546, 'predictions_19': -0.2782951415110554, 'predictions_20': 1.2470508123020512, 'predictions_21': 1.049830317708393, 'predictions_22': 2.102971796648596, 'predictions_23': 0.2851979247349288, 'predictions_24': -0.0006702647871052775, 'predictions_25': -0.11420596882801563, 'predictions_26': 1.0834575497816143, 'predictions_27': 0.8164104508549398, 'predictions_28': 0.06634783513626033, 'predictions_29': 0.10518170393387423, 'predictions_30': 0.05948635171854934, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.008846265655878418, 'predictions_1': 1.071493056050533, 'predictions_2': 0.0241002358002765, 'predictions_3': -0.2914009217752569, 'predictions_4': -0.1844514405858182, 'predictions_5': 0.09688586653158524, 'predictions_6': 0.1189004872794518, 'predictions_7': 0.019812317046639417, 'predictions_8': -0.0841879790447643, 'predictions_9': 0.0689671067023492, 'predictions_10': 0.057123796305462, 'predictions_11': 0.018751811843757425, 'predictions_12': -0.19278774516524225, 'predictions_13': 0.5521382975001031, 'predictions_14': -0.1961614983559944, 'predictions_15': 0.3352816348185536, 'predictions_16': -0.009197695434128215, 'predictions_17': -0.0600167757501572, 'predictions_18': 0.27488314466683056, 'predictions_19': -0.35962747336476697, 'predictions_20': 1.2317107478669351, 'predictions_21': 0.05530975604521487, 'predictions_22': 2.382011011440535, 'predictions_23': 0.33824065775317486, 'predictions_24': 0.3498540690011901, 'predictions_25': 0.1739274660593352, 'predictions_26': 1.160333734158511, 'predictions_27': 1.033879786485623, 'predictions_28': 0.08158573366246898, 'predictions_29': 0.10563970622307337, 'predictions_30': -0.04267793892712356, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.004750001129083867, 'predictions_1': 3.2244208404334374, 'predictions_2': 0.01622715285279811, 'predictions_3': -0.817260302999862, 'predictions_4': -0.09736090983332732, 'predictions_5': 0.07881792915896496, 'predictions_6': 0.24070898834769355, 'predictions_7': 0.05001221074373508, 'predictions_8': -0.2567854774979608, 'predictions_9': 0.03063087506346955, 'predictions_10': 0.05499599036837444, 'predictions_11': -0.015303644634305683, 'predictions_12': -0.14884606737286166, 'predictions_13': 0.8519672928318166, 'predictions_14': 0.09824149785766935, 'predictions_15': 0.26921023748269235, 'predictions_16': -0.010848751281971217, 'predictions_17': -0.11619083730523652, 'predictions_18': -0.17527472428145596, 'predictions_19': -0.5874677933384177, 'predictions_20': -0.3990904299729458, 'predictions_21': 2.2068328291797936, 'predictions_22': -1.932202847332452, 'predictions_23': -0.3152964245377162, 'predictions_24': 0.7834452171983805, 'predictions_25': 0.2512128072560273, 'predictions_26': -0.6206434154152907, 'predictions_27': 0.08708205787374604, 'predictions_28': 0.040648951231987765, 'predictions_29': 0.06879586583909683, 'predictions_30': 0.043515107484221424, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': -0.011027810657660349, 'predictions_1': -0.5337191669392997, 'predictions_2': -0.0026033241771052282, 'predictions_3': -0.2382336633486158, 'predictions_4': 0.8615636351404935, 'predictions_5': 0.059347121609268944, 'predictions_6': 0.14253423982272048, 'predictions_7': 1.4462830393121449, 'predictions_8': -0.06536550111076092, 'predictions_9': 0.12249420022849346, 'predictions_10': -0.040845467674169966, 'predictions_11': 0.03619973926410233, 'predictions_12': -0.14839345664605622, 'predictions_13': -0.38765958181699983, 'predictions_14': 0.45137385893985227, 'predictions_15': 0.4818261473751218, 'predictions_16': 0.005229328958126197, 'predictions_17': -0.14927291449462546, 'predictions_18': 0.12257473692108792, 'predictions_19': 0.5775523654869467, 'predictions_20': 1.4945158847763393, 'predictions_21': -0.11572127634540279, 'predictions_22': 1.2803791500605577, 'predictions_23': 0.2519454034779557, 'predictions_24': 0.12639705427540554, 'predictions_25': 0.20374090734634412, 'predictions_26': 0.9872077234715891, 'predictions_27': 1.1931782325388345, 'predictions_28': 0.07647609206107736, 'predictions_29': 0.017535160650109134, 'predictions_30': -0.011152247353355573, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': -0.009236814888869137, 'predictions_1': -0.08974532751806996, 'predictions_2': -0.005072446447076212, 'predictions_3': -0.49454476931590674, 'predictions_4': -0.14583165960960504, 'predictions_5': 0.037743362980294, 'predictions_6': -0.09071218034159645, 'predictions_7': -2.076157655204495, 'predictions_8': 0.6915530135596496, 'predictions_9': 0.015305309316520455, 'predictions_10': -0.05407297473599998, 'predictions_11': -0.01202689608724274, 'predictions_12': -0.37048240770136764, 'predictions_13': -1.1222567180136822, 'predictions_14': 0.037999849333804875, 'predictions_15': 0.05179781531623324, 'predictions_16': -0.009442169563784072, 'predictions_17': -0.3518926772423797, 'predictions_18': -0.18168464537700557, 'predictions_19': -0.246308669315598, 'predictions_20': -1.8215267653197431, 'predictions_21': -0.16464307910939846, 'predictions_22': -2.294068720859334, 'predictions_23': -0.3304406806357679, 'predictions_24': -0.8059935139116144, 'predictions_25': -0.15473187742974112, 'predictions_26': -0.44492987082868113, 'predictions_27': -1.706574981012038, 'predictions_28': 0.009928350750753007, 'predictions_29': -0.005531569126011125, 'predictions_30': 0.03400893184303606, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.002893918337539625, 'predictions_1': 0.6953965947651528, 'predictions_2': -0.013300368855470382, 'predictions_3': -0.15693491782098012, 'predictions_4': 0.4052561639196121, 'predictions_5': -0.01785344804083238, 'predictions_6': 0.19406598570732034, 'predictions_7': 1.5796202341560919, 'predictions_8': 0.28954821935673325, 'predictions_9': -0.215897797520852, 'predictions_10': 0.05835282036206641, 'predictions_11': 0.03331176153763488, 'predictions_12': -0.10112958834294049, 'predictions_13': 0.3947745629125056, 'predictions_14': 0.22909135741673778, 'predictions_15': 0.473005657256218, 'predictions_16': -0.009633689567643305, 'predictions_17': -0.09362381604913257, 'predictions_18': 0.14969971629912343, 'predictions_19': -0.1688864705396212, 'predictions_20': 1.3001215347067874, 'predictions_21': -0.21918668227485943, 'predictions_22': 1.3437058168797267, 'predictions_23': 0.3124907025891718, 'predictions_24': 0.14131080537131419, 'predictions_25': 0.2243700411172835, 'predictions_26': 0.9296630907535046, 'predictions_27': 0.41471504174869356, 'predictions_28': 0.020173572275052214, 'predictions_29': 0.04820465228613692, 'predictions_30': -0.020545384469295942, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.0019388150699810927, 'predictions_1': 0.9223705632364128, 'predictions_2': -0.012007685883043798, 'predictions_3': -0.31903137131372966, 'predictions_4': 0.628481905867853, 'predictions_5': -0.06149389221971728, 'predictions_6': 0.07061611794203079, 'predictions_7': 1.3823056189088423, 'predictions_8': -0.133343124664483, 'predictions_9': 0.11603949252367812, 'predictions_10': 0.21857476218484376, 'predictions_11': 0.015902798791774055, 'predictions_12': 0.2913666065699202, 'predictions_13': 0.9315294837553827, 'predictions_14': 0.277372510153019, 'predictions_15': -0.5071083100622337, 'predictions_16': -0.009631147961094873, 'predictions_17': 0.23976024706824375, 'predictions_18': -0.20540519019181294, 'predictions_19': -0.2450413530123813, 'predictions_20': 1.1789579806256083, 'predictions_21': -1.5177833757024324, 'predictions_22': 1.4604002248277352, 'predictions_23': 0.27531878725283415, 'predictions_24': -1.352094841156462, 'predictions_25': 0.16870219247146698, 'predictions_26': 1.2263320807717468, 'predictions_27': 0.8656450275023648, 'predictions_28': 0.04415827467622267, 'predictions_29': 0.049210669003044466, 'predictions_30': 0.03485239596130599, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.003483875604996794, 'predictions_1': 0.9677217395705439, 'predictions_2': -0.01375116195045965, 'predictions_3': -0.3148394441913672, 'predictions_4': -0.2574040676795255, 'predictions_5': 0.07782351238517007, 'predictions_6': 0.09223237164727777, 'predictions_7': 1.359163521325679, 'predictions_8': -0.10520478897286097, 'predictions_9': 0.051820926250002466, 'predictions_10': 0.15651454755052202, 'predictions_11': 0.012354841533717503, 'predictions_12': 0.29314938008831337, 'predictions_13': 1.0134451429783053, 'predictions_14': 0.07334166731849916, 'predictions_15': -0.5580245806930221, 'predictions_16': -0.009637538822743917, 'predictions_17': -0.12931888564696647, 'predictions_18': -0.08985648327837921, 'predictions_19': -0.2838831478457971, 'predictions_20': 1.197739882302604, 'predictions_21': -0.14264086768498266, 'predictions_22': 2.4168915798709034, 'predictions_23': 0.35060520926622657, 'predictions_24': -0.243435195670719, 'predictions_25': 0.15680747277488985, 'predictions_26': 1.2012113470638528, 'predictions_27': 0.9897751319349664, 'predictions_28': 0.05573907097988011, 'predictions_29': 0.06252860717834312, 'predictions_30': -0.05792966463337761, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.015122615065552805, 'predictions_1': -1.1167653489947622, 'predictions_2': -0.008012147012472742, 'predictions_3': -0.20874221256644707, 'predictions_4': 0.4252072619730782, 'predictions_5': 0.0038900875799020296, 'predictions_6': 0.1140119630004244, 'predictions_7': 1.1987104625838227, 'predictions_8': -0.0802347059616203, 'predictions_9': 0.14227487864929314, 'predictions_10': 0.061570146412656145, 'predictions_11': -0.0013235117361348366, 'predictions_12': 0.22496427871452854, 'predictions_13': 0.6826705611065566, 'predictions_14': 0.331084179340632, 'predictions_15': 0.2325873510907064, 'predictions_16': -0.005890948415758354, 'predictions_17': 0.23108082656181192, 'predictions_18': 0.08866538976848874, 'predictions_19': 0.5251741787977718, 'predictions_20': 1.5307505517513718, 'predictions_21': -0.07014338016238107, 'predictions_22': 1.8024293796373567, 'predictions_23': 0.3420427562962711, 'predictions_24': -0.3356060347979862, 'predictions_25': 0.08823113765567157, 'predictions_26': 0.9993112240252872, 'predictions_27': 1.1583364010362838, 'predictions_28': 0.05818942683648322, 'predictions_29': -0.010171113593323115, 'predictions_30': 0.017500344327137828, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.00510007523648401, 'predictions_1': -1.8438586845336085, 'predictions_2': 0.07034588660781765, 'predictions_3': -0.6334911581627888, 'predictions_4': 0.9114601232034509, 'predictions_5': 0.015641139153578926, 'predictions_6': 0.3391581513516312, 'predictions_7': 0.015993612058473987, 'predictions_8': 0.4979057135726034, 'predictions_9': 0.14140896753303245, 'predictions_10': 0.03348118561743431, 'predictions_11': 0.018019313387541973, 'predictions_12': -0.1851865976812716, 'predictions_13': 0.18463673035754868, 'predictions_14': 0.3321862529567762, 'predictions_15': 0.4582953091852766, 'predictions_16': -0.023872509230380146, 'predictions_17': -0.05714457269664822, 'predictions_18': 0.1677010761064405, 'predictions_19': 0.6590215547332258, 'predictions_20': -0.4470726570372422, 'predictions_21': -1.2957188152033094, 'predictions_22': -0.49568168502602117, 'predictions_23': -0.5319175224432703, 'predictions_24': 0.8792904089758667, 'predictions_25': -0.16764333932557407, 'predictions_26': -0.5006140094263773, 'predictions_27': -0.559662593948684, 'predictions_28': 0.009575432219475658, 'predictions_29': 0.03620587401831965, 'predictions_30': -0.022617768077518082, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': -0.0035275503865915213, 'predictions_1': -1.7572879984733045, 'predictions_2': 0.02112961345058588, 'predictions_3': -0.5373126141152578, 'predictions_4': 0.8167634172202621, 'predictions_5': -0.04568688093881375, 'predictions_6': 0.25612518616907237, 'predictions_7': 1.6585574657259259, 'predictions_8': 0.5708485569128593, 'predictions_9': -0.2579041111541445, 'predictions_10': -0.02303431468406031, 'predictions_11': 0.0294850340796527, 'predictions_12': -0.20150167754729115, 'predictions_13': -0.3122660955180186, 'predictions_14': 0.08946441512158586, 'predictions_15': 0.19599051996984723, 'predictions_16': -0.007048153187921441, 'predictions_17': -0.10443059840913398, 'predictions_18': 0.14693551914712497, 'predictions_19': -0.2646088763947528, 'predictions_20': -0.14423328554566225, 'predictions_21': -1.096484854939204, 'predictions_22': -0.5409155996124657, 'predictions_23': -0.2125528115678197, 'predictions_24': 0.2028301059851276, 'predictions_25': 0.15076353496237724, 'predictions_26': 1.863813974679114, 'predictions_27': 1.2625204294969739, 'predictions_28': -0.009542569876103744, 'predictions_29': 0.08892200769099384, 'predictions_30': 0.016344768954324324, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.009144654047150176, 'predictions_1': 0.3616068989438007, 'predictions_2': -0.009713127697925978, 'predictions_3': -0.25503380890174077, 'predictions_4': -0.08841376708924753, 'predictions_5': 0.12905387860630704, 'predictions_6': 0.09872550234229752, 'predictions_7': 1.5461380270279617, 'predictions_8': 0.5934142135359506, 'predictions_9': 0.04672843933802434, 'predictions_10': 0.017982350366210965, 'predictions_11': 0.011836524186964618, 'predictions_12': -0.09329132650766998, 'predictions_13': 0.9816979966957412, 'predictions_14': -0.26131805604494435, 'predictions_15': 0.2573728246698596, 'predictions_16': -0.009616853447343936, 'predictions_17': -0.11778440476199589, 'predictions_18': 0.19894108953925974, 'predictions_19': -0.28976560140618507, 'predictions_20': 1.145755494068452, 'predictions_21': 0.19170884942775918, 'predictions_22': 1.751619931359333, 'predictions_23': 0.31591084941785597, 'predictions_24': -0.9883146017669252, 'predictions_25': 0.3832169744602564, 'predictions_26': 1.3459027320296548, 'predictions_27': 1.0895032649194054, 'predictions_28': 0.054326669111151096, 'predictions_29': 0.11224841710144848, 'predictions_30': -0.01934243236389702, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.05414474380600745, 'predictions_1': -1.149372263278166, 'predictions_2': -0.0034542658503694534, 'predictions_3': -0.11226127026369175, 'predictions_4': -0.2765479593192703, 'predictions_5': 0.057605254673602974, 'predictions_6': 0.04807218948118946, 'predictions_7': 1.627632661158546, 'predictions_8': 0.23594239851080898, 'predictions_9': 0.08102266882022441, 'predictions_10': -0.035797597595999694, 'predictions_11': -0.006940512375528646, 'predictions_12': -0.10341465914545066, 'predictions_13': 0.27134162901025793, 'predictions_14': -0.4589675261254597, 'predictions_15': 0.16906923946657362, 'predictions_16': -0.005805030106413082, 'predictions_17': -0.11670739934889805, 'predictions_18': 0.270439579413901, 'predictions_19': 0.2757024597749045, 'predictions_20': 1.2679444783850085, 'predictions_21': -1.2185063190204835, 'predictions_22': 2.6862730600162457, 'predictions_23': 0.45079291995440945, 'predictions_24': -0.8576927701312551, 'predictions_25': 0.1825880636881889, 'predictions_26': 0.9481775337394789, 'predictions_27': 1.3019845783662138, 'predictions_28': 0.03309325718132554, 'predictions_29': 0.037279537345320794, 'predictions_30': 0.030849407280271066, 'predictions_31': 1.5201632854544105}\n",
|
|
"{'predictions_0': 0.026701912078513444, 'predictions_1': -0.016049183561005216, 'predictions_2': -0.026512557715316794, 'predictions_3': -0.33992007086017256, 'predictions_4': -0.3231034954783173, 'predictions_5': 0.020522588667874812, 'predictions_6': -0.09818245278711138, 'predictions_7': -1.9632581054922957, 'predictions_8': 0.2796715168175009, 'predictions_9': 0.025963248780199805, 'predictions_10': -0.13243884691329014, 'predictions_11': -0.007600341414574132, 'predictions_12': -0.3505614312588073, 'predictions_13': -0.8449241022454159, 'predictions_14': -0.0623541831245574, 'predictions_15': 0.11533014973600747, 'predictions_16': 0.008322220108907262, 'predictions_17': -0.02930862278171467, 'predictions_18': 0.02496960430979726, 'predictions_19': 0.3997160251519232, 'predictions_20': -2.0119476119311948, 'predictions_21': -0.3601922717542553, 'predictions_22': -2.240466883625807, 'predictions_23': -0.24430626245778664, 'predictions_24': -0.732571668183472, 'predictions_25': -0.14435610495492934, 'predictions_26': -0.4186367055351456, 'predictions_27': -1.7801593987201698, 'predictions_28': 0.014498054148804375, 'predictions_29': -0.10768829118597369, 'predictions_30': -0.02172472974992555, 'predictions_31': 1.5201632854544105}\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"\u001b[2m\u001b[36m(pid=1492090)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1492090)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1492090)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.Float64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1492090)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1492090)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/dask/dataframe/backends.py:181: FutureWarning: pandas.UInt64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1492090)\u001b[0m _numeric_index_types = (pd.Int64Index, pd.Float64Index, pd.UInt64Index)\n",
|
|
"\u001b[2m\u001b[36m(pid=1492090)\u001b[0m /home/ubuntu/ray/venv/lib/python3.8/site-packages/xgboost/compat.py:31: FutureWarning: pandas.Int64Index is deprecated and will be removed from pandas in a future version. Use pandas.Index with the appropriate dtype instead.\n",
|
|
"\u001b[2m\u001b[36m(pid=1492090)\u001b[0m from pandas import MultiIndex, Int64Index\n"
|
|
]
|
|
}
|
|
],
|
|
"source": [
|
|
"predict_lightgbm(result)"
|
|
]
|
|
}
|
|
],
|
|
"metadata": {
|
|
"jupytext": {
|
|
"cell_metadata_filter": "-all",
|
|
"main_language": "python",
|
|
"notebook_metadata_filter": "-all"
|
|
},
|
|
"kernelspec": {
|
|
"display_name": "Python 3.8.10 ('venv': venv)",
|
|
"language": "python",
|
|
"name": "python3"
|
|
},
|
|
"language_info": {
|
|
"codemirror_mode": {
|
|
"name": "ipython",
|
|
"version": 3
|
|
},
|
|
"file_extension": ".py",
|
|
"mimetype": "text/x-python",
|
|
"name": "python",
|
|
"nbconvert_exporter": "python",
|
|
"pygments_lexer": "ipython3",
|
|
"version": "3.8.10"
|
|
},
|
|
"vscode": {
|
|
"interpreter": {
|
|
"hash": "3c0d54d489a08ae47a06eae2fd00ff032d6cddb527c382959b7b2575f6a8167f"
|
|
}
|
|
}
|
|
},
|
|
"nbformat": 4,
|
|
"nbformat_minor": 5
|
|
}
|