mirror of
https://github.com/vale981/emacs-jupyter
synced 2025-03-05 23:41:38 -05:00
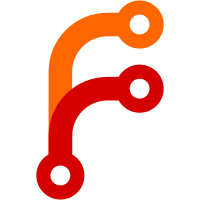
- `jupyter-client.el` only contains client related code - `jupyter-kernel-manager` related code is placed in `jupyter-kernel-manager.el` - Socket creating functions and generating connection info plist function are placed in `jupyter-connection.el`. This also contains the `jupyter-connection` class. - Kernelspec related functions are placed in `jupyter-kernelspec.el` - Move general utility functions and variables requires necessary for `jupyter` into `jupyter-base.el`. This also contains the `jupyter-session` and `jupyter-request` struct definitions.
50 lines
1.7 KiB
EmacsLisp
50 lines
1.7 KiB
EmacsLisp
(require 'json)
|
|
|
|
(defvar jupyter--kernelspec-dirs nil
|
|
"An alist matching kernel names to their kernelspec
|
|
directories.")
|
|
|
|
(defun jupyter-available-kernelspecs (&optional force-new)
|
|
"Get the available kernelspecs.
|
|
Return an alist mapping kernel names to their kernelspec
|
|
directories. The alist is formed by a call to the shell command
|
|
|
|
jupyter kernelspec list
|
|
|
|
By default the available kernelspecs are cached. To force an
|
|
update of the cached kernelspecs set FORCE-NEW to a non-nil
|
|
value."
|
|
(unless (or jupyter--kernelspec-dirs force-new)
|
|
(setq jupyter--kernelspec-dirs
|
|
(mapcar (lambda (s) (let ((s (split-string s " " 'omitnull)))
|
|
(cons (car s) (cadr s))))
|
|
(seq-subseq
|
|
(split-string
|
|
(shell-command-to-string "jupyter kernelspec list")
|
|
"\n" 'omitnull "[ \t]+")
|
|
1))))
|
|
jupyter--kernelspec-dirs)
|
|
|
|
(defun jupyter-find-kernelspec (prefix)
|
|
"Find the first kernelspec for the kernel that matches PREFIX.
|
|
From the available kernelspecs returned by
|
|
`jupyter-available-kernelspecs' return a cons cell
|
|
|
|
(KERNEL-NAME . PLIST)
|
|
|
|
where KERNEL-NAME is the name of the kernel that begins with
|
|
PREFIX and PLIST is the kernelspec PLIST read from the
|
|
\"kernel.json\" file in the kernel's kernelspec directory."
|
|
(when prefix
|
|
(let ((kname-path (cl-find-if
|
|
(lambda (s) (string-prefix-p prefix (car s)))
|
|
(jupyter-available-kernelspecs)))
|
|
(json-object-type 'plist)
|
|
(json-array-type 'list)
|
|
(json-false nil))
|
|
(when kname-path
|
|
(cons (car kname-path)
|
|
(json-read-file
|
|
(expand-file-name "kernel.json" (cdr kname-path))))))))
|
|
|
|
(provide 'jupyter-kernelspec)
|