mirror of
https://github.com/vale981/emacs-ipython-notebook
synced 2025-03-05 09:01:40 -05:00
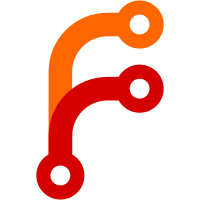
M-x customize-group RET ein Toggle Ein:Polymode Avoid trying to emulate jump-to-definition, eldoc, and autocompletion functionalities that Elpy will always do better. Fixes #497 #482 #418
76 lines
3.1 KiB
EmacsLisp
76 lines
3.1 KiB
EmacsLisp
(eval-when-compile (require 'cl))
|
||
(require 'ert)
|
||
|
||
(require 'ein-core)
|
||
|
||
;; Generic getter
|
||
|
||
(defmacro eintest:generic-getter-should-return-nil (func)
|
||
"In an \"empty\" context, generic getter should return nil."
|
||
`(ert-deftest ,(intern (format "%s--nil name" func)) ()
|
||
(with-temp-buffer
|
||
(should (not (,func))))))
|
||
|
||
(eintest:generic-getter-should-return-nil ein:get-url-or-port)
|
||
(eintest:generic-getter-should-return-nil ein:get-notebook)
|
||
(eintest:generic-getter-should-return-nil ein:get-kernel)
|
||
(eintest:generic-getter-should-return-nil ein:get-cell-at-point)
|
||
(eintest:generic-getter-should-return-nil ein:get-traceback-data)
|
||
|
||
|
||
|
||
;;; File name translation
|
||
|
||
;; Requiring `tramp' during (inside of) tests yields error from
|
||
;; MuMaMo. Although I don't understand the reason, requiring it
|
||
;; before running tests workarounds this problem.
|
||
(require 'tramp)
|
||
|
||
(ert-deftest ein:filename-translations-from-to-tramp ()
|
||
;; I really don't understand this https://github.com/magit/with-editor/issues/29
|
||
:expected-result (if (>= emacs-major-version 26) :failed :passed)
|
||
(loop with ein:filename-translations =
|
||
`((8888 . ,(ein:tramp-create-filename-translator "HOST" "USER")))
|
||
with filename = "/file/name"
|
||
for port in '(7777 8888) ; check for the one w/o translation
|
||
for emacs-filename = (ein:filename-from-python port filename)
|
||
do (message "emacs-filename = %s" emacs-filename)
|
||
do (should
|
||
(equal (ein:filename-to-python port emacs-filename)
|
||
filename))))
|
||
|
||
(ert-deftest ein:filename-translations-to-from-tramp ()
|
||
:expected-result (if (>= emacs-major-version 26) :failed :passed)
|
||
(loop with ein:filename-translations =
|
||
`((8888 . ,(ein:tramp-create-filename-translator "HOST" "USER")))
|
||
with filename = "/USER@HOST:/filename"
|
||
for port in '(8888)
|
||
do (should
|
||
(equal (ein:filename-from-python
|
||
port (ein:filename-to-python port filename))
|
||
filename))))
|
||
|
||
(ert-deftest ein:filename-to-python-tramp ()
|
||
:expected-result (if (>= emacs-major-version 26) :failed :passed)
|
||
(let* ((port 8888)
|
||
(ein:filename-translations
|
||
`((,port . ,(ein:tramp-create-filename-translator "DUMMY")))))
|
||
(loop with python-filename = "/file/name"
|
||
for emacs-filename in '("/scpc:HOST:/file/name"
|
||
"/USER@HOST:/file/name")
|
||
do (should
|
||
(equal (ein:filename-to-python port emacs-filename)
|
||
python-filename)))
|
||
;; Error: Not a Tramp file name: /file/name
|
||
(should-error (ein:filename-to-python port "/file/name"))))
|
||
|
||
(ert-deftest ein:filename-from-python-tramp ()
|
||
:expected-result (if (>= emacs-major-version 26) :failed :passed)
|
||
(loop with ein:filename-translations =
|
||
`((8888 . ,(ein:tramp-create-filename-translator "HOST" "USER")))
|
||
with python-filename = "/file/name"
|
||
for emacs-filename in '("/USER@HOST:/file/name" "/file/name")
|
||
for port in '(8888 7777)
|
||
do (should
|
||
(equal (ein:filename-from-python port python-filename)
|
||
emacs-filename))))
|