mirror of
https://github.com/vale981/apollo-server
synced 2025-03-17 08:16:40 -04:00
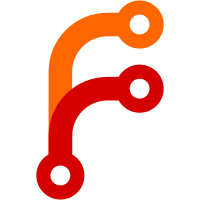
* Setup prettier and precommit hooks * Format code with prettier * Use husky because it works... * Move prettier config to .prettierrc file * Implement fixing markdown file formatting when running lint-fix script * Format markdown files * Add .json file formatting * Fixes json file formatting * Add pretteir linting step * Remove tslint * Use gitignore for prettier * Fix linting errors * Ignore submodule folder
74 lines
2 KiB
TypeScript
74 lines
2 KiB
TypeScript
import AdonisContext from '@adonisjs/framework/src/Context';
|
|
import {
|
|
GraphQLOptions,
|
|
HttpQueryError,
|
|
runHttpQuery,
|
|
} from 'apollo-server-core';
|
|
import * as GraphiQL from 'apollo-server-module-graphiql';
|
|
|
|
export interface AdonisGraphQLOptionsFunction {
|
|
(ctx: AdonisContext): GraphQLOptions | Promise<GraphQLOptions>;
|
|
}
|
|
|
|
export interface AdonisHandler {
|
|
(req: any, next): void;
|
|
}
|
|
|
|
export function graphqlAdonis(
|
|
options: GraphQLOptions | AdonisGraphQLOptionsFunction,
|
|
): AdonisHandler {
|
|
if (!options) {
|
|
throw new Error('Apollo Server requires options.');
|
|
}
|
|
if (arguments.length > 1) {
|
|
throw new Error(
|
|
`Apollo Server expects exactly one argument, got ${arguments.length}`,
|
|
);
|
|
}
|
|
return (ctx: AdonisContext): Promise<void> => {
|
|
const { request, response } = ctx;
|
|
const method = request.method();
|
|
const query = method === 'POST' ? request.post() : request.get();
|
|
return runHttpQuery([ctx], {
|
|
method,
|
|
options,
|
|
query,
|
|
}).then(
|
|
gqlResponse => {
|
|
response.json(gqlResponse);
|
|
},
|
|
(error: HttpQueryError) => {
|
|
if ('HttpQueryError' !== error.name) {
|
|
throw error;
|
|
}
|
|
if (error.headers) {
|
|
Object.keys(error.headers).forEach(header => {
|
|
response.header(header, error.headers[header]);
|
|
});
|
|
}
|
|
response.status(error.statusCode).send(error.message);
|
|
},
|
|
);
|
|
};
|
|
}
|
|
|
|
export interface AdonisGraphiQLOptionsFunction {
|
|
(ctx: AdonisContext): GraphiQL.GraphiQLData | Promise<GraphiQL.GraphiQLData>;
|
|
}
|
|
|
|
export function graphiqlAdonis(
|
|
options: GraphiQL.GraphiQLData | AdonisGraphiQLOptionsFunction,
|
|
) {
|
|
return (ctx: AdonisContext): Promise<void> => {
|
|
const { request, response } = ctx;
|
|
const query = request.get();
|
|
return GraphiQL.resolveGraphiQLString(query, options, ctx).then(
|
|
graphiqlString => {
|
|
response.type('text/html').send(graphiqlString);
|
|
},
|
|
(error: HttpQueryError) => {
|
|
response.status(500).send(error.message);
|
|
},
|
|
);
|
|
};
|
|
}
|