mirror of
https://github.com/vale981/Vulcan
synced 2025-03-10 12:36:39 -04:00
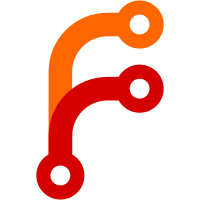
This commit touch a lot of lines of code with the goal to be more rigorous about JavaScript code conventions defined in the `.jshintrc`. Some modification: * Add a list of used global symbols in the corresponding section of `.jshintrc` * Use local variables instead of global in a lot of places where the keyword `var` was mistakenly forgotten * Add missing semi-colons after instructions * Add new lines at the end of files * Remove trailing whitespaces * Use newer name of some Meteor APIs, eg `addFiles` instead of `add_files` * Add missing `break` statements in `switch` blocks * Use `===` instead of `==` and `!==` instead of `!=` * Remove unused variables This commit should also fix a few bugs due to this lack of rigor. One example of that was the test `typeof navElements === "array"` that was never true because in JavaScript, `typeof [] === "object"`, we replaced this test by the `_.isArray` method provided by underscore. It might also fix some potential collision related to global variables. There is still plenty of work until Telescope code base passes jsHint validation, but at least this commit is a step in the right direction.
90 lines
2.7 KiB
JavaScript
90 lines
2.7 KiB
JavaScript
defaultFrequency = 7;
|
|
defaultPosts = 5;
|
|
|
|
getCampaignPosts = function (postsCount) {
|
|
|
|
// look for last scheduled campaign in the database
|
|
var lastCampaign = SyncedCron._collection.findOne({name: 'Schedule newsletter'}, {sort: {finishedAt: -1}, limit: 1});
|
|
|
|
// if there is a last campaign use its date, else default to posts from the last 7 days
|
|
var lastWeek = moment().subtract(7, 'days').toDate();
|
|
var after = (typeof lastCampaign !== 'undefined') ? lastCampaign.finishedAt : lastWeek
|
|
|
|
var params = Posts.getSubParams({
|
|
view: 'campaign',
|
|
limit: postsCount,
|
|
after: after
|
|
});
|
|
return Posts.find(params.find, params.options).fetch();
|
|
};
|
|
|
|
buildCampaign = function (postsArray) {
|
|
var postsHTML = '', subject = '';
|
|
|
|
// 1. Iterate through posts and pass each of them through a handlebars template
|
|
postsArray.forEach(function (post, index) {
|
|
if(index > 0)
|
|
subject += ', ';
|
|
|
|
subject += post.title;
|
|
|
|
var postUser = Meteor.users.findOne(post.userId);
|
|
|
|
// the naked post object as stored in the database is missing a few properties, so let's add them
|
|
var properties = _.extend(post, {
|
|
authorName: Users.getAuthorName(post),
|
|
postLink: Posts.getLink(post),
|
|
profileUrl: Users.getProfileUrl(postUser),
|
|
postPageLink: Posts.getPageUrl(post),
|
|
date: moment(post.postedAt).format("MMMM D YYYY")
|
|
});
|
|
|
|
if (post.body)
|
|
properties.body = marked(Telescope.utils.trimWords(post.body, 20)).replace('<p>', '').replace('</p>', ''); // remove p tags
|
|
|
|
if(post.url)
|
|
properties.domain = Telescope.utils.getDomain(post.url);
|
|
|
|
postsHTML += getEmailTemplate('emailPostItem')(properties);
|
|
});
|
|
|
|
// 2. Wrap posts HTML in digest template
|
|
var digestHTML = getEmailTemplate('emailDigest')({
|
|
siteName: Settings.get('title'),
|
|
date: moment().format("dddd, MMMM Do YYYY"),
|
|
content: postsHTML
|
|
});
|
|
|
|
// 3. wrap digest HTML in email wrapper template
|
|
var emailHTML = buildEmailTemplate(digestHTML);
|
|
|
|
var campaign = {
|
|
postIds: _.pluck(postsArray, '_id'),
|
|
subject: Telescope.utils.trimWords(subject, 15),
|
|
html: emailHTML
|
|
};
|
|
|
|
return campaign;
|
|
};
|
|
|
|
scheduleNextCampaign = function (isTest) {
|
|
isTest = !! isTest;
|
|
var posts = getCampaignPosts(Settings.get('postsPerNewsletter', defaultPosts));
|
|
if(!!posts.length){
|
|
return scheduleCampaign(buildCampaign(posts), isTest);
|
|
}else{
|
|
var result = 'No posts to schedule today…';
|
|
return result;
|
|
}
|
|
};
|
|
|
|
Meteor.methods({
|
|
sendCampaign: function () {
|
|
if(Users.is.adminById(this.userId))
|
|
return scheduleNextCampaign(false);
|
|
},
|
|
testCampaign: function () {
|
|
if(Users.is.adminById(this.userId))
|
|
return scheduleNextCampaign(true);
|
|
}
|
|
});
|