mirror of
https://github.com/vale981/Vulcan
synced 2025-03-08 19:11:38 -05:00
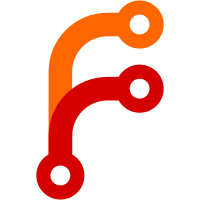
1. make intl fields use [IntlValue] and [IntlValueInput] types 2. add isIntlData marker to fields storing intl data 3. only validate intl fields that are marked as required 4. remove all instance of `__typename` from documents in edit forms
68 lines
No EOL
1.5 KiB
JavaScript
68 lines
No EOL
1.5 KiB
JavaScript
import SimpleSchema from 'simpl-schema';
|
|
|
|
export const Locales = [];
|
|
|
|
export const registerLocale = locale => {
|
|
Locales.push(locale);
|
|
}
|
|
|
|
/*
|
|
|
|
Look for type name in a few different places
|
|
Note: look into simplifying this
|
|
|
|
*/
|
|
export const isIntlField = fieldSchema => {
|
|
return fieldSchema.intl;
|
|
}
|
|
|
|
/*
|
|
|
|
Generate custom IntlString SimpleSchema type
|
|
|
|
*/
|
|
export const getIntlString = () => {
|
|
|
|
const schema = {
|
|
locale: {
|
|
type: String,
|
|
optional: true,
|
|
},
|
|
value: {
|
|
type: String,
|
|
optional: true,
|
|
}
|
|
};
|
|
|
|
const IntlString = new SimpleSchema(schema);
|
|
IntlString.name = 'IntlString';
|
|
return IntlString;
|
|
}
|
|
|
|
/*
|
|
|
|
Custom validation function to check for required locales
|
|
|
|
See https://github.com/aldeed/simple-schema-js#custom-field-validation
|
|
|
|
*/
|
|
export const validateIntlField = function () {
|
|
let errors = [];
|
|
|
|
// go through locales to check which one are required
|
|
const requiredLocales = Locales.filter(locale => locale.required);
|
|
|
|
requiredLocales.forEach((locale, index) => {
|
|
const strings = this.value;
|
|
const hasString = strings && strings.some(s => s && s.locale === locale.id && s.value);
|
|
if (!hasString) {
|
|
const originalFieldName = this.key.replace('_intl', '');
|
|
errors.push({ id: 'errors.required', path: `${this.key}.${index}`, properties: { name: originalFieldName, locale: locale.id }});
|
|
}
|
|
});
|
|
|
|
if (errors.length > 0) {
|
|
// hack to work around the fact that custom validation function can only return a single string
|
|
return `intlError|${JSON.stringify(errors)}`;
|
|
}
|
|
} |